mirror of
https://github.com/matrix-org/sliding-sync.git
synced 2025-03-10 13:37:11 +00:00
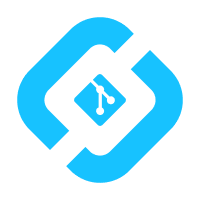
- `Conn`s now expose a direct `OnUpdate(caches.Update)` function for updates which concern a specific device ID. - Add a bitset in `DeviceData` to indicate if the OTK or fallback keys were changed. - Pass through the affected `DeviceID` in `pubsub.V2DeviceData` updates. - Remove `DeviceDataTable.SelectFrom` as it was unused. - Refactor how the poller invokes `OnE2EEData`: it now only does this if there are changes to OTK counts and/or fallback key types and/or device lists, and _only_ sends those fields, setting the rest to the zero value. - Remove noisy logging. - Add `caches.DeviceDataUpdate` which has no data but serves to wake-up the long poller. - Only send OTK counts / fallback key types when they have changed, not constantly. This matches the behaviour described in MSC3884 The entire flow now looks like: - Poller notices a diff against in-memory version of otk count and invokes `OnE2EEData` - Handler updates device data table, bumps the changed bit for otk count. - Other handler gets the pubsub update, directly finds the `Conn` based on the `DeviceID`. Invokes `OnUpdate(caches.DeviceDataUpdate)` - This update is handled by the E2EE extension which then pulls the data out from the database and returns it. - On initial connections, all OTK / fallback data is returned.
58 lines
1.1 KiB
Go
58 lines
1.1 KiB
Go
package internal
|
|
|
|
import "testing"
|
|
|
|
func TestDeviceDataBitset(t *testing.T) {
|
|
testCases := []struct {
|
|
get func() DeviceData
|
|
fallbackSet bool
|
|
otkSet bool
|
|
}{
|
|
{
|
|
get: func() DeviceData {
|
|
var dd DeviceData
|
|
dd.SetFallbackKeysChanged()
|
|
return dd
|
|
},
|
|
fallbackSet: true,
|
|
otkSet: false,
|
|
},
|
|
{
|
|
get: func() DeviceData {
|
|
var dd DeviceData
|
|
dd.SetOTKCountChanged()
|
|
return dd
|
|
},
|
|
fallbackSet: false,
|
|
otkSet: true,
|
|
},
|
|
{
|
|
get: func() DeviceData {
|
|
var dd DeviceData
|
|
dd.SetFallbackKeysChanged()
|
|
dd.SetOTKCountChanged()
|
|
return dd
|
|
},
|
|
fallbackSet: true,
|
|
otkSet: true,
|
|
},
|
|
{
|
|
get: func() DeviceData {
|
|
var dd DeviceData
|
|
return dd
|
|
},
|
|
fallbackSet: false,
|
|
otkSet: false,
|
|
},
|
|
}
|
|
for _, tc := range testCases {
|
|
dd := tc.get()
|
|
if dd.FallbackKeysChanged() != tc.fallbackSet {
|
|
t.Errorf("%v : wrong fallback value, want %v", dd, tc.fallbackSet)
|
|
}
|
|
if dd.OTKCountChanged() != tc.otkSet {
|
|
t.Errorf("%v : wrong OTK value, want %v", dd, tc.otkSet)
|
|
}
|
|
}
|
|
}
|