mirror of
https://github.com/matrix-org/matrix-hookshot.git
synced 2025-03-10 13:17:08 +00:00
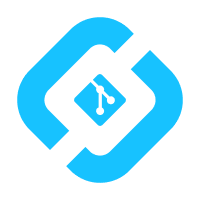
* Add support for checking connection grants * Make this less noisy * Remove import * Trying to generic-ize the grant system * Implement for Figma * More grant reformatting * Add tests * changelog * Fix todo * Refactor grants * Add logging to grant checkers * Ensure we provide a sender
36 lines
965 B
TypeScript
36 lines
965 B
TypeScript
import { IntentMock } from "./IntentMock";
|
|
|
|
export class AppserviceMock {
|
|
// eslint-disable-next-line @typescript-eslint/no-explicit-any
|
|
public readonly intentMap = new Map<string, any>();
|
|
public readonly botIntent = IntentMock.create(`@bot:example.com`);
|
|
public namespace = "@hookshot_";
|
|
|
|
static create(){
|
|
// eslint-disable-next-line @typescript-eslint/no-explicit-any
|
|
return new this() as any;
|
|
}
|
|
|
|
get botUserId() {
|
|
return this.botIntent.userId;
|
|
}
|
|
|
|
get botClient() {
|
|
return this.botIntent.underlyingClient;
|
|
}
|
|
|
|
public getIntentForUserId(userId: string) {
|
|
let intent = this.intentMap.get(userId);
|
|
if (intent) {
|
|
return intent;
|
|
}
|
|
intent = IntentMock.create(userId);
|
|
this.intentMap.set(userId, intent);
|
|
return intent;
|
|
}
|
|
|
|
public isNamespacedUser(userId: string) {
|
|
return userId.startsWith(this.namespace);
|
|
}
|
|
}
|