mirror of
https://github.com/element-hq/element-x-ios.git
synced 2025-03-10 13:37:11 +00:00
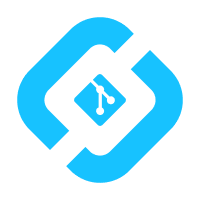
* New LICENSE-COMMERCIAL file * Apply dual licenses: AGPL + Element Commercial to file headers * Update README with dual licensing
37 lines
1023 B
Swift
37 lines
1023 B
Swift
//
|
|
// Copyright 2023, 2024 New Vector Ltd.
|
|
//
|
|
// SPDX-License-Identifier: AGPL-3.0-only OR LicenseRef-Element-Commercial
|
|
// Please see LICENSE files in the repository root for full details.
|
|
//
|
|
|
|
import Combine
|
|
|
|
/// A wrapper of CurrentValueSubject.
|
|
/// The purpose of this type is to remove the possibility to send new values on the underlying subject.
|
|
struct CurrentValuePublisher<Output, Failure: Error>: Publisher {
|
|
private let subject: CurrentValueSubject<Output, Failure>
|
|
|
|
init(_ subject: CurrentValueSubject<Output, Failure>) {
|
|
self.subject = subject
|
|
}
|
|
|
|
init(_ value: Output) {
|
|
self.init(CurrentValueSubject(value))
|
|
}
|
|
|
|
func receive<S>(subscriber: S) where S: Subscriber, Failure == S.Failure, Output == S.Input {
|
|
subject.receive(subscriber: subscriber)
|
|
}
|
|
|
|
var value: Output {
|
|
subject.value
|
|
}
|
|
}
|
|
|
|
extension CurrentValueSubject {
|
|
func asCurrentValuePublisher() -> CurrentValuePublisher<Output, Failure> {
|
|
.init(self)
|
|
}
|
|
}
|