mirror of
https://github.com/element-hq/element-x-ios.git
synced 2025-03-10 21:39:12 +00:00
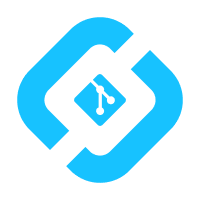
* Use the new TimelineMediaPreview modifier on the room and pinned timeline screens. * Use the same presentation logic for all timeline media previews. * Fix a bug with the detection of the timeline end. * Send pagination requests from the media preview screen. * Add SwiftLint to the Danger workflow (it is no longer installed on the runner). * Put SwiftLint back on all of the GitHub runners too. * Set the function_parameter_count lint rule to 10. * Make sure to clean-up any previews when the coordinator is done. * Handle the viewInRoomTimeline action more appropriately.
132 lines
4.9 KiB
Swift
132 lines
4.9 KiB
Swift
//
|
|
// Copyright 2023, 2024 New Vector Ltd.
|
|
//
|
|
// SPDX-License-Identifier: AGPL-3.0-only OR LicenseRef-Element-Commercial
|
|
// Please see LICENSE files in the repository root for full details.
|
|
//
|
|
|
|
import Combine
|
|
import Foundation
|
|
import MatrixRustSDK
|
|
|
|
enum TimelineKind: Equatable {
|
|
case live
|
|
case detached
|
|
case pinned
|
|
|
|
enum MediaPresentation { case roomScreenLive, roomScreenDetached, pinnedEventsScreen, mediaFilesScreen }
|
|
case media(MediaPresentation)
|
|
}
|
|
|
|
enum TimelineFocus {
|
|
case live
|
|
case eventID(String)
|
|
case pinned
|
|
}
|
|
|
|
enum TimelineAllowedMessageType {
|
|
case audio, file, image, video
|
|
}
|
|
|
|
enum TimelineProxyError: Error {
|
|
case sdkError(Error)
|
|
|
|
case failedRedacting
|
|
case failedPaginatingEndReached
|
|
}
|
|
|
|
// sourcery: AutoMockable
|
|
protocol TimelineProxyProtocol {
|
|
var timelineProvider: TimelineProviderProtocol { get }
|
|
|
|
func subscribeForUpdates() async
|
|
|
|
func fetchDetails(for eventID: String)
|
|
|
|
func messageEventContent(for timelineItemID: TimelineItemIdentifier) async -> RoomMessageEventContentWithoutRelation?
|
|
|
|
func retryDecryption(sessionIDs: [String]?) async
|
|
|
|
func paginateBackwards(requestSize: UInt16) async -> Result<Void, TimelineProxyError>
|
|
func paginateForwards(requestSize: UInt16) async -> Result<Void, TimelineProxyError>
|
|
|
|
func edit(_ eventOrTransactionID: TimelineItemIdentifier.EventOrTransactionID,
|
|
newContent: EditedContent) async -> Result<Void, TimelineProxyError>
|
|
|
|
func redact(_ eventOrTransactionID: TimelineItemIdentifier.EventOrTransactionID,
|
|
reason: String?) async -> Result<Void, TimelineProxyError>
|
|
|
|
func pin(eventID: String) async -> Result<Bool, TimelineProxyError>
|
|
|
|
func unpin(eventID: String) async -> Result<Bool, TimelineProxyError>
|
|
|
|
// MARK: - Sending
|
|
|
|
func sendAudio(url: URL,
|
|
audioInfo: AudioInfo,
|
|
caption: String?,
|
|
requestHandle: @MainActor (SendAttachmentJoinHandleProtocol) -> Void) async -> Result<Void, TimelineProxyError>
|
|
|
|
func sendFile(url: URL,
|
|
fileInfo: FileInfo,
|
|
caption: String?,
|
|
requestHandle: @MainActor (SendAttachmentJoinHandleProtocol) -> Void) async -> Result<Void, TimelineProxyError>
|
|
|
|
func sendImage(url: URL,
|
|
thumbnailURL: URL,
|
|
imageInfo: ImageInfo,
|
|
caption: String?,
|
|
requestHandle: @MainActor (SendAttachmentJoinHandleProtocol) -> Void) async -> Result<Void, TimelineProxyError>
|
|
|
|
func sendLocation(body: String,
|
|
geoURI: GeoURI,
|
|
description: String?,
|
|
zoomLevel: UInt8?,
|
|
assetType: AssetType?) async -> Result<Void, TimelineProxyError>
|
|
|
|
func sendVideo(url: URL,
|
|
thumbnailURL: URL,
|
|
videoInfo: VideoInfo,
|
|
caption: String?,
|
|
requestHandle: @MainActor (SendAttachmentJoinHandleProtocol) -> Void) async -> Result<Void, TimelineProxyError>
|
|
|
|
func sendVoiceMessage(url: URL,
|
|
audioInfo: AudioInfo,
|
|
waveform: [UInt16],
|
|
requestHandle: @MainActor (SendAttachmentJoinHandleProtocol) -> Void) async -> Result<Void, TimelineProxyError>
|
|
|
|
func sendReadReceipt(for eventID: String, type: ReceiptType) async -> Result<Void, TimelineProxyError>
|
|
|
|
func sendMessageEventContent(_ messageContent: RoomMessageEventContentWithoutRelation) async -> Result<Void, TimelineProxyError>
|
|
|
|
func sendMessage(_ message: String,
|
|
html: String?,
|
|
inReplyToEventID: String?,
|
|
intentionalMentions: IntentionalMentions) async -> Result<Void, TimelineProxyError>
|
|
|
|
func toggleReaction(_ reaction: String, to eventID: TimelineItemIdentifier.EventOrTransactionID) async -> Result<Void, TimelineProxyError>
|
|
|
|
func createPoll(question: String, answers: [String], pollKind: Poll.Kind) async -> Result<Void, TimelineProxyError>
|
|
|
|
func editPoll(original eventID: String,
|
|
question: String,
|
|
answers: [String],
|
|
pollKind: Poll.Kind) async -> Result<Void, TimelineProxyError>
|
|
|
|
func endPoll(pollStartID: String, text: String) async -> Result<Void, TimelineProxyError>
|
|
|
|
func sendPollResponse(pollStartID: String, answers: [String]) async -> Result<Void, TimelineProxyError>
|
|
|
|
func getLoadedReplyDetails(eventID: String) async -> Result<InReplyToDetails, TimelineProxyError>
|
|
|
|
func buildMessageContentFor(_ message: String,
|
|
html: String?,
|
|
intentionalMentions: Mentions) -> RoomMessageEventContentWithoutRelation
|
|
}
|
|
|
|
extension TimelineProxyProtocol {
|
|
func retryDecryption() async {
|
|
await retryDecryption(sessionIDs: nil)
|
|
}
|
|
}
|