mirror of
https://github.com/element-hq/element-x-ios.git
synced 2025-03-10 21:39:12 +00:00
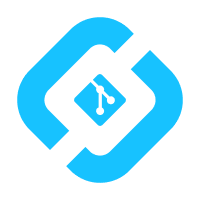
* Fix Timeline on Xcode 14/iOS 16 Raise requirement to iOS 16+ Reduce pagination jumping. Sonarcloud fixes. Fix verification test. Adopt if let optional { syntax. * Remove unused ScrollViewReader The ScrollViewReader didn't appear to change the behaviour. * Fix warnings on Run Scripts. Run script build phase 'SwiftLint' will be run during every build because it does not specify any outputs. To address this warning, either add output dependencies to the script phase, or configure it to run in every build by unchecking "Based on dependency analysis" in the script phase.
275 lines
7.0 KiB
Ruby
275 lines
7.0 KiB
Ruby
require 'yaml'
|
|
require_relative 'changelog'
|
|
|
|
before_all do
|
|
xcversion(version: "~> 14.0")
|
|
end
|
|
|
|
lane :alpha do
|
|
|
|
config_xcodegen_alpha()
|
|
|
|
xcodegen(spec: "project.yml")
|
|
|
|
provisioning_profile_name = "ElementX PR Ad Hoc"
|
|
bundle_identifier = "io.element.elementx.pr"
|
|
code_signing_identity = "Apple Distribution: Vector Creations Limited (7J4U792NQT)"
|
|
|
|
update_code_signing_settings(
|
|
use_automatic_signing: false,
|
|
bundle_identifier: bundle_identifier,
|
|
profile_name: provisioning_profile_name,
|
|
code_sign_identity: code_signing_identity
|
|
)
|
|
|
|
get_provisioning_profile(
|
|
app_identifier: bundle_identifier,
|
|
provisioning_name: provisioning_profile_name,
|
|
ignore_profiles_with_different_name: true,
|
|
adhoc: true
|
|
)
|
|
|
|
version = ENV["GITHUB_PR_NUMBER"]
|
|
|
|
update_app_icon(caption_text: "PR #{version}")
|
|
|
|
bump_build_number()
|
|
|
|
build_ios_app(
|
|
scheme: "ElementX",
|
|
clean: true,
|
|
export_method: "ad-hoc",
|
|
output_directory: "build",
|
|
export_options: {
|
|
provisioningProfiles: {
|
|
bundle_identifier => provisioning_profile_name,
|
|
}
|
|
}
|
|
)
|
|
|
|
upload_to_diawi()
|
|
|
|
end
|
|
|
|
lane :github_release do
|
|
build_adhoc()
|
|
|
|
upload_to_diawi()
|
|
|
|
release_to_github()
|
|
|
|
prepare_next_release()
|
|
|
|
upload_dsyms_to_sentry()
|
|
end
|
|
|
|
lane :app_store_release do
|
|
build_release()
|
|
|
|
release_to_github()
|
|
|
|
prepare_next_release()
|
|
|
|
upload_dsyms_to_sentry()
|
|
end
|
|
|
|
|
|
lane :build_adhoc do
|
|
bump_build_number()
|
|
|
|
build_ios_app(
|
|
scheme: "ElementX",
|
|
clean: true,
|
|
export_method: "ad-hoc",
|
|
output_directory: "build",
|
|
export_options: {
|
|
provisioningProfiles: {
|
|
"io.element.elementx" => "ElementX Ad Hoc",
|
|
}
|
|
}
|
|
)
|
|
end
|
|
|
|
lane :build_release do
|
|
bump_build_number()
|
|
|
|
build_ios_app(
|
|
scheme: "ElementX",
|
|
clean: true,
|
|
export_method: "app-store",
|
|
output_directory: "build",
|
|
xcargs: "-allowProvisioningUpdates",
|
|
)
|
|
end
|
|
|
|
lane :unit_tests do
|
|
run_tests(
|
|
scheme: "UnitTests"
|
|
)
|
|
|
|
slather(
|
|
cobertura_xml: true,
|
|
output_directory: "./fastlane/test_output",
|
|
proj: "ElementX.xcodeproj",
|
|
scheme: "UnitTests",
|
|
)
|
|
end
|
|
|
|
lane :ui_tests do
|
|
run_tests(
|
|
scheme: "UITests",
|
|
devices: ["iPhone 13 Pro Max (15.5)", "iPad (9th generation) (15.5)"],
|
|
ensure_devices_found: true,
|
|
prelaunch_simulator: true,
|
|
result_bundle: true
|
|
)
|
|
|
|
slather(
|
|
cobertura_xml: true,
|
|
output_directory: "./fastlane/test_output",
|
|
proj: "ElementX.xcodeproj",
|
|
scheme: "UITests",
|
|
binary_basename: "ElementX.app"
|
|
)
|
|
end
|
|
|
|
lane :integration_tests do
|
|
run_tests(
|
|
scheme: "IntegrationTests",
|
|
devices: ["iPhone 13 Pro"],
|
|
ensure_devices_found: true,
|
|
)
|
|
end
|
|
|
|
private_lane :bump_build_number do
|
|
# Increment build number to current date
|
|
build_number = Time.now.strftime("%Y%m%d%H%M")
|
|
increment_build_number(build_number: build_number)
|
|
end
|
|
|
|
private_lane :config_xcodegen_alpha do
|
|
target_file_path = "../ElementX/SupportingFiles/target.yml"
|
|
data = YAML.load_file target_file_path
|
|
data["targets"]["ElementX"]["settings"]["base"]["PRODUCT_NAME"] = "ElementX PR"
|
|
data["targets"]["ElementX"]["settings"]["base"]["PRODUCT_BUNDLE_IDENTIFIER"] = "io.element.elementx.pr"
|
|
File.open(target_file_path, 'w') { |f| YAML.dump(data, f) }
|
|
end
|
|
|
|
desc "Upload IPA to Diawi"
|
|
private_lane :upload_to_diawi do
|
|
api_token = ENV["DIAWI_API_TOKEN"]
|
|
UI.user_error!("Invalid Diawi API token.") unless !api_token.to_s.empty?
|
|
|
|
# Upload to Diawi
|
|
diawi(
|
|
token: api_token,
|
|
wall_of_apps: false,
|
|
file: lane_context[SharedValues::IPA_OUTPUT_PATH]
|
|
)
|
|
|
|
# Get the Diawi link from Diawi action shared value
|
|
diawi_link = lane_context[SharedValues::UPLOADED_FILE_LINK_TO_DIAWI]
|
|
UI.command_output("Diawi link: " + diawi_link.to_s)
|
|
|
|
# Generate the Diawi QR code file link
|
|
diawi_app_id = URI(diawi_link).path.split('/').last
|
|
diawi_qr_code_link = "https://www.diawi.com/qrcode/link/#{diawi_app_id}"
|
|
|
|
# Set "DIAWI_FILE_LINK" to GitHub environment variables for Github actions
|
|
sh("echo DIAWI_FILE_LINK=#{diawi_link} >> $GITHUB_ENV")
|
|
sh("echo DIAWI_QR_CODE_LINK=#{diawi_qr_code_link} >> $GITHUB_ENV")
|
|
end
|
|
|
|
desc "Create GitHub Release"
|
|
private_lane :release_to_github do
|
|
api_token = ENV["GITHUB_TOKEN"]
|
|
UI.user_error!("Invalid GitHub API token.") unless !api_token.to_s.empty?
|
|
|
|
# Get the Diawi link from Diawi action shared value
|
|
diawi_link = lane_context[SharedValues::UPLOADED_FILE_LINK_TO_DIAWI]
|
|
|
|
release_version = get_version_number()
|
|
|
|
changes = export_version_changes(version: release_version)
|
|
|
|
description = ""
|
|
if diawi_link.nil?
|
|
description = "#{changes}"
|
|
else
|
|
# Generate the Diawi QR code file link
|
|
diawi_app_id = URI(diawi_link).path.split('/').last
|
|
diawi_qr_code_link = "https://www.diawi.com/qrcode/link/#{diawi_app_id}"
|
|
|
|
"[iOS AdHoc Release - Diawi Link](#{diawi_link})
|
|

|
|
#{changes}"
|
|
end
|
|
|
|
github_release = set_github_release(
|
|
repository_name: "vector-im/element-x-ios",
|
|
api_token: api_token,
|
|
name: release_version,
|
|
tag_name: release_version,
|
|
is_generate_release_notes: false,
|
|
description: description
|
|
)
|
|
|
|
end
|
|
|
|
private_lane :prepare_next_release do
|
|
target_file_path = "../ElementX/SupportingFiles/target.yml"
|
|
xcode_project_file_path = "../ElementX.xcodeproj"
|
|
|
|
data = YAML.load_file target_file_path
|
|
current_version = data["targets"]["ElementX"]["settings"]["base"]["MARKETING_VERSION"]
|
|
new_version = current_version.next
|
|
|
|
# Bump the patch version. The empty string after -i is so that sed doesn't
|
|
# create a backup file on macOS
|
|
sh("sed -i '' 's/#{current_version}/#{new_version}/g' #{target_file_path}")
|
|
|
|
xcodegen(spec: "project.yml")
|
|
|
|
sh("git add #{target_file_path} #{xcode_project_file_path}")
|
|
|
|
sh("git commit -m 'Prepare next release'")
|
|
end
|
|
|
|
private_lane :export_version_changes do |options|
|
|
Dir.chdir("..") do
|
|
Changelog.update_topmost_section(version: options[:version], additional_entries: {})
|
|
Changelog.extract_first_section
|
|
end
|
|
end
|
|
|
|
private_lane :update_app_icon do |options|
|
|
caption_text = options[:caption_text]
|
|
UI.user_error!("Invalid caption text.") unless !caption_text.to_s.empty?
|
|
|
|
Dir.glob("../ElementX/Resources/Assets.xcassets/AppIcon.appiconset/**/*.png") do |file_name|
|
|
# Change the icons color
|
|
sh("convert '#{file_name}' -modulate 100,100,200 '#{file_name}'")
|
|
|
|
caption_width = sh("identify -format %w '#{file_name}'")
|
|
caption_height = file_name.end_with?("@2x.png") ? 60 : 30
|
|
if caption_width.to_i > caption_height*2 then
|
|
|
|
# Add a label on top
|
|
sh("convert -background '#0008' -fill white -gravity center -size '#{caption_width}'x'#{caption_height}' caption:'#{caption_text}' '#{file_name}' +swap -gravity south -composite '#{file_name}'")
|
|
end
|
|
end
|
|
end
|
|
|
|
private_lane :upload_dsyms_to_sentry do
|
|
auth_token = ENV["SENTRY_AUTH_TOKEN"]
|
|
UI.user_error!("Invalid Sentry Auth token.") unless !auth_token.to_s.empty?
|
|
|
|
sentry_upload_dif(
|
|
auth_token: auth_token,
|
|
org_slug: 'element',
|
|
project_slug: 'elementx',
|
|
url: 'https://sentry.tools.element.io/',
|
|
path: './build/ElementX.app.dSYM.zip',
|
|
)
|
|
end
|