mirror of
https://github.com/element-hq/element-x-ios.git
synced 2025-03-11 13:59:13 +00:00
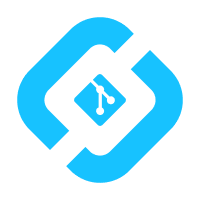
* Disable image and document picker integration tests as they randomly fail to load and are flakey. * Delete any pre-existing log files * Various tracing tweaks and fixes: - delete the custom tracing log levels as we can't control their ouput - implement comparable on them - change default levels only if the new chosen level increases their verbosity * Make logging targets mandatory and fix their logging levels * Switch back to using the `run_tests` reset simulator flag as `fastlane snapshot reset_simulators` was too generic and slow * Switch all integration test taps to `tapCenter` (nee forceTap) after noticing missed taps on CI. * Make the logging file prefix explicit, let the main app not use one. * Rename tracing configuration `target` to `currentTarget`
45 lines
2.8 KiB
Swift
45 lines
2.8 KiB
Swift
//
|
|
// Copyright 2022-2024 New Vector Ltd.
|
|
//
|
|
// SPDX-License-Identifier: AGPL-3.0-only
|
|
// Please see LICENSE in the repository root for full details.
|
|
//
|
|
|
|
import XCTest
|
|
|
|
@testable import ElementX
|
|
|
|
class TracingConfigurationTests: XCTestCase {
|
|
func testConfiguration() { // swiftlint:disable line_length
|
|
var filter = TracingConfiguration(logLevel: .error, currentTarget: "tests", filePrefix: nil).filter
|
|
|
|
XCTAssertEqual(filter, "hyper=warn,matrix_sdk_ffi=info,matrix_sdk::client=trace,matrix_sdk_crypto=debug,matrix_sdk_crypto::olm::account=trace,matrix_sdk::oidc=trace,matrix_sdk::http_client=debug,matrix_sdk::sliding_sync=info,matrix_sdk_base::sliding_sync=info,matrix_sdk_ui::timeline=info,tests=error")
|
|
|
|
filter = TracingConfiguration(logLevel: .warn, currentTarget: "tests", filePrefix: nil).filter
|
|
|
|
XCTAssertEqual(filter, "hyper=warn,matrix_sdk_ffi=info,matrix_sdk::client=trace,matrix_sdk_crypto=debug,matrix_sdk_crypto::olm::account=trace,matrix_sdk::oidc=trace,matrix_sdk::http_client=debug,matrix_sdk::sliding_sync=info,matrix_sdk_base::sliding_sync=info,matrix_sdk_ui::timeline=info,tests=warn")
|
|
|
|
filter = TracingConfiguration(logLevel: .info, currentTarget: "tests", filePrefix: nil).filter
|
|
|
|
XCTAssertEqual(filter, "hyper=warn,matrix_sdk_ffi=info,matrix_sdk::client=trace,matrix_sdk_crypto=debug,matrix_sdk_crypto::olm::account=trace,matrix_sdk::oidc=trace,matrix_sdk::http_client=debug,matrix_sdk::sliding_sync=info,matrix_sdk_base::sliding_sync=info,matrix_sdk_ui::timeline=info,tests=info")
|
|
|
|
filter = TracingConfiguration(logLevel: .debug, currentTarget: "tests", filePrefix: nil).filter
|
|
|
|
XCTAssertEqual(filter, "hyper=warn,matrix_sdk_ffi=debug,matrix_sdk::client=trace,matrix_sdk_crypto=debug,matrix_sdk_crypto::olm::account=trace,matrix_sdk::oidc=trace,matrix_sdk::http_client=debug,matrix_sdk::sliding_sync=debug,matrix_sdk_base::sliding_sync=debug,matrix_sdk_ui::timeline=debug,tests=debug")
|
|
|
|
filter = TracingConfiguration(logLevel: .trace, currentTarget: "tests", filePrefix: nil).filter
|
|
|
|
XCTAssertEqual(filter, "hyper=warn,matrix_sdk_ffi=trace,matrix_sdk::client=trace,matrix_sdk_crypto=trace,matrix_sdk_crypto::olm::account=trace,matrix_sdk::oidc=trace,matrix_sdk::http_client=trace,matrix_sdk::sliding_sync=trace,matrix_sdk_base::sliding_sync=trace,matrix_sdk_ui::timeline=trace,tests=trace")
|
|
} // swiftlint:enable line_length
|
|
|
|
func testLevelOrdering() {
|
|
var logLevels: [TracingConfiguration.LogLevel] = [.info, .error, .trace, .debug, .warn]
|
|
|
|
XCTAssertEqual(logLevels.sorted(), [.error, .warn, .info, .debug, .trace])
|
|
|
|
logLevels = [.warn, .error, .debug, .trace, .info, .error]
|
|
|
|
XCTAssertEqual(logLevels.sorted(), [.error, .error, .warn, .info, .debug, .trace])
|
|
}
|
|
}
|