mirror of
https://github.com/element-hq/element-x-ios.git
synced 2025-03-10 21:39:12 +00:00
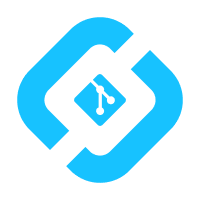
* provider can now check the current session * More testable code * created the test condition * it works but not always not sure why, need to dig deeper * sadly we need to use textkit 1 to solve this issue * removed developer option screen test * this experimental solution kinda works but I need a way to pill recomputation is weird * format * display improvement * better and faster solution * pilished the code * better coloring * swift format * just need to solve the caching issue * fix caching issue * tests done! * changelog * pr comments addressed * all pr comments addressed * docs * line lenght * updated tests and fixed a parsing permalink issue * MentionBuilder * pr comments * swiftformat * code blocks should not have links * Update ElementX/Sources/FlowCoordinators/RoomFlowCoordinator.swift Co-authored-by: Doug <6060466+pixlwave@users.noreply.github.com> * Update ElementX/Sources/Services/Client/ClientProxy.swift Co-authored-by: Doug <6060466+pixlwave@users.noreply.github.com> * Update UnitTests/Sources/AttributedStringBuilderTests.swift Co-authored-by: Doug <6060466+pixlwave@users.noreply.github.com> * Update ElementX/Sources/UITests/UITestsAppCoordinator.swift Co-authored-by: Doug <6060466+pixlwave@users.noreply.github.com> * Update ElementX/Sources/Other/Pills/PillAttachmentViewProvider.swift Co-authored-by: Doug <6060466+pixlwave@users.noreply.github.com> * Update ElementX/Sources/Other/Pills/MentionBuilder.swift Co-authored-by: Doug <6060466+pixlwave@users.noreply.github.com> * pr comments * swiftformat --------- Co-authored-by: Doug <6060466+pixlwave@users.noreply.github.com>
67 lines
2.7 KiB
Swift
67 lines
2.7 KiB
Swift
//
|
|
// Copyright 2023 New Vector Ltd
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
//
|
|
|
|
import SwiftUI
|
|
import SwiftUIIntrospect
|
|
import UIKit
|
|
|
|
final class PillAttachmentViewProvider: NSTextAttachmentViewProvider {
|
|
private weak var messageTextView: MessageTextView?
|
|
|
|
// MARK: - Override
|
|
|
|
override init(textAttachment: NSTextAttachment, parentView: UIView?, textLayoutManager: NSTextLayoutManager?, location: NSTextLocation) {
|
|
super.init(textAttachment: textAttachment, parentView: parentView, textLayoutManager: textLayoutManager, location: location)
|
|
|
|
// Keep a reference to the parent text view for size adjustments and pills flushing.
|
|
messageTextView = parentView?.superview as? MessageTextView
|
|
tracksTextAttachmentViewBounds = true
|
|
}
|
|
|
|
@MainActor
|
|
override func loadView() {
|
|
super.loadView()
|
|
|
|
guard let textAttachmentData = (textAttachment as? PillTextAttachment)?.pillData else {
|
|
MXLog.failure("[PillAttachmentViewProvider]: attachment is missing data or not of expected class")
|
|
return
|
|
}
|
|
|
|
let viewModel: PillContext
|
|
let imageProvider: ImageProviderProtocol?
|
|
if ProcessInfo.isXcodePreview || ProcessInfo.isRunningTests {
|
|
// The mock viewModel simulates the loading logic for testing purposes
|
|
viewModel = PillContext.mock(type: .loadUser)
|
|
imageProvider = MockMediaProvider()
|
|
} else if let roomContext = messageTextView?.roomContext {
|
|
viewModel = PillContext(roomContext: roomContext, data: textAttachmentData)
|
|
imageProvider = roomContext.imageProvider
|
|
} else {
|
|
MXLog.failure("[PillAttachmentViewProvider]: missing room context")
|
|
return
|
|
}
|
|
|
|
let view = PillView(imageProvider: imageProvider, viewModel: viewModel) { [weak self] in
|
|
self?.messageTextView?.invalidateTextAttachmentsDisplay(update: true)
|
|
}
|
|
let controller = UIHostingController(rootView: view)
|
|
controller.view.backgroundColor = .clear
|
|
// This allows the text view to handle it as a link
|
|
controller.view.isUserInteractionEnabled = false
|
|
self.view = controller.view
|
|
}
|
|
}
|