mirror of
https://github.com/element-hq/element-x-ios.git
synced 2025-03-10 21:39:12 +00:00
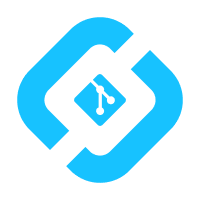
* MediaUploadingPreprocessor - Prevent images without any GPS metadata from being changed * Add support for sending images * Add support for sending video, audio and file attachments * Rename MediaPickerPreview to MediaUploadPreview * Move media uploading to the MediaUploadPreviewScreen, add waiting indicators and error handling * Add support for pasting and drag&dropping in media for upload * Adopt new media picker source UI, remove developer flag * Set minimum heights for timeline loadable images * Fix invalid camera picker file names * Fix flakey MediaUploadingPreprocessor image tests, improve gps metadata stripping * UITests: Update existing screenshots and add new step for the room attachment picker * Switch all github action runners to macos-13 * Cleanup enter key and paste message composer handlers
87 lines
4.2 KiB
Swift
87 lines
4.2 KiB
Swift
//
|
|
// Copyright 2022 New Vector Ltd
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
//
|
|
|
|
import Foundation
|
|
import SwiftUI
|
|
|
|
struct ImageRoomTimelineView: View {
|
|
@EnvironmentObject private var context: RoomScreenViewModel.Context
|
|
let timelineItem: ImageRoomTimelineItem
|
|
|
|
var body: some View {
|
|
TimelineStyler(timelineItem: timelineItem) {
|
|
LoadableImage(mediaSource: timelineItem.source,
|
|
blurhash: timelineItem.blurhash,
|
|
imageProvider: context.imageProvider) {
|
|
placeholder
|
|
}
|
|
.frame(maxHeight: min(300, max(100, timelineItem.height ?? .infinity)))
|
|
.aspectRatio(timelineItem.aspectRatio, contentMode: .fit)
|
|
}
|
|
}
|
|
|
|
var placeholder: some View {
|
|
ZStack {
|
|
Rectangle()
|
|
.foregroundColor(.element.systemGray6)
|
|
.opacity(0.3)
|
|
|
|
ProgressView(L10n.commonLoading)
|
|
.frame(maxWidth: .infinity)
|
|
}
|
|
}
|
|
}
|
|
|
|
struct ImageRoomTimelineView_Previews: PreviewProvider {
|
|
static let viewModel = RoomScreenViewModel.mock
|
|
|
|
static var previews: some View {
|
|
body.environmentObject(viewModel.context)
|
|
body.timelineStyle(.plain).environmentObject(viewModel.context)
|
|
}
|
|
|
|
static var body: some View {
|
|
VStack(spacing: 20.0) {
|
|
ImageRoomTimelineView(timelineItem: ImageRoomTimelineItem(id: UUID().uuidString,
|
|
body: "Some image",
|
|
timestamp: "Now",
|
|
isOutgoing: false,
|
|
isEditable: false,
|
|
sender: .init(id: "Bob"),
|
|
source: MediaSourceProxy(url: .picturesDirectory, mimeType: "image/png")))
|
|
|
|
ImageRoomTimelineView(timelineItem: ImageRoomTimelineItem(id: UUID().uuidString,
|
|
body: "Some other image",
|
|
timestamp: "Now",
|
|
isOutgoing: false,
|
|
isEditable: false,
|
|
sender: .init(id: "Bob"),
|
|
source: MediaSourceProxy(url: .picturesDirectory, mimeType: "image/png")))
|
|
|
|
ImageRoomTimelineView(timelineItem: ImageRoomTimelineItem(id: UUID().uuidString,
|
|
body: "Blurhashed image",
|
|
timestamp: "Now",
|
|
isOutgoing: false,
|
|
isEditable: false,
|
|
sender: .init(id: "Bob"),
|
|
source: MediaSourceProxy(url: .picturesDirectory, mimeType: "image/gif"),
|
|
aspectRatio: 0.7,
|
|
blurhash: "L%KUc%kqS$RP?Ks,WEf8OlrqaekW",
|
|
contentType: .gif))
|
|
}
|
|
}
|
|
}
|